About This Course
Learn C++, a high-performance programming language used in the world’s most exciting engineering jobs — from self-driving cars and robotics, to web browsers, media platforms, servers, and even video games. Get hands-on experience by coding five real-world projects. Learn to build a route planner using OpenStreetMap data, write a process monitor for your computer, and implement your own smart pointers.
Finally, showcase all your newfound skills by building a multithreaded traffic simulator and coding your own C++ application.
Prerequisite Knowledge: To optimize your chances of success in the C++ Nanodegree program, we recommend intermediate knowledge of any programming language.
Certificate
Learning Objectives
C++ is a compiled, high-performance language. Robots, automobiles, and embedded software all depend on C++ for speed of execution. This program is designed to turn software engineers into C++ developers. You will use C++ to develop object-oriented programs, to manage memory and system resources, and to implement parallel programming.
Requirements
- There is no application. This Nanodegree program accepts everyone, regardless of experience and specific background.
Target Audience
- This program is right for you if you’re an intermediate-level programmer familiar with functions and classes who wants to become a C++ developer or pursue a career in robotics software, IoT, mobile communications, video game development, operating systems, networking, AI, embedded systems, and more.
Curriculum
414 Lessons
Welcome to the C++ Developer Nanodegree Program
Introduction to C++
01. C++0:40Preview
02. C++ History1:44
03. C++ Today0:27
04. Modern C++1:05
05. C++ Core Guidlines0:40
05.2 C++ Core Guidlines3:50
06. Standard Library
07. Compilation
08. Build Tools
09. Editors0:55
10. Style1:06
Workspaces
01. Introduction to Workspaces
02. Notebook Workspaces
03. Terminal Workspaces
Welcome to Bootcamp AI
01. What It Takes
Connecting with Your Community
Access the Career Portal
How Do I Find Time for My Nanodegree?
Welcome
Learn basic C++ syntax, functions, containers, and compiling
and linking with multiple files. Use OpenStreetMap and the 2D visualization library IO2D to build a route planner that displays a path between two points on a map.
02. Talks About the C++ Language00:1:48
02.2 Talks About the C++ Language00:1:37
02.3 Talks About the C++ Language00:00:34
02.4 Talks About the C++ Language00:1:43
02.5 Talks About the C++ Language0:42
03. How to Learn C++1:16
03.2 How to Learn C++1:27
04. The Core Guidelines0:40
04.2 The Core Guidelines3:50
Introduction to the C++ Language
01. Intro0:57
02. CODE Write and Run Your First C++ Program
03. Compiled Languages vs Scripted Languages3:35
03.2 Compiled Languages vs Scripted Languages1:46
04. C++ Output and Language Basics
05. CODE Send Output to the Console
06. How to Store Data
07. Introduces C++ Types1:09
08. Primitive Variable Types
09. What is a Vector0:47
10. C++ Vectors
11. C++ Comments0:59
12. Using Auto
13. CODE Store a Grid in Your Program
14. Getting Ready for Printing
15. Working with Vectors00:00:00
16. For Loops00:00:00
17. Functions00:00:00
18. CODE Print the Board00:00:00
19. If Statements and While Loops00:00:00
20. Reading from a File00:00:00
21. CODE Read the Board from a File00:00:00
22. Processing Strings00:00:00
23. Adding Data to a Vector00:00:00
24. CODE Parse Lines from the File00:00:00
25. CODE Use the ParseLine Function00:00:00
26. Formatting the Printed Board
27. CODE Formatting the Printed Board00:00:00
28. CODE Store the Board using the State Enum00:00:00
29. Great Work!0:22
A Search
01. Intro0:43
02. Motion Planning2:06
03. Maze0:33
03.2 Maze0:16
04. Maze 21:12
04.2 Maze 20:10
05. Coding the Shortest Path Algorithm2:43
06. A Search8:27
07. Lesson Code Structure1:54
07. Lesson Code Structure
08. CODE Starting A Search00:00:00
09. CODE Writing the A Heuristic00:00:28
09.2 CODE Writing the A Heuristic00:00:00
10. Pass by Reference in C++00:00:00
11. CODE Adding Nodes to the Open Vector00:00:00
12. CODE Initialize the Open Vector00:00:00
13. CODE Create a Comparison Function00:00:45
13.2 CODE Create a Comparison Function00:00:00
14. CODE Write a While Loop for the A Algorithm00:00:00
15. CODE Check for Valid Neighbors00:00:20
15.2 CODE Check for Valid Neighbors00:00:00
16. Constants00:00:00
17. CODE Expand the A Search to Neighbors00:00:00
18. Arrays1:16
19. CODE: Adding a Start and End to the Board00:00:00
20. Congratulations!!0:35
21. How to Become More Proficient at C++0:49
Writing Larger Programs
01. Intro0:51
02. Header Files
03. Using Multiple Files00:00:00
04. Build Systems0:52
05. CMake and Make00:00:00
06. References00:00:00
07. Pointers00:00:00
09. References vs Pointers
10. Dictionary Example in Code00:00:00
11. Classes and Object-Oriented Programming00:00:00
12. Classes and OOP Continued00:00:00
13. Outro0:21
Extending the OpenStreetMap Data Model
01. Intro00:00:35
02. The OpenStreetMap Project
02. The OpenStreetMap Project
03. IO2D Starter Code2:19
03.2 IO2D Starter Code2:48
03.3 IO2D Starter Code1:08
03.4 IO2D Starter Code1:27
03.5 IO2D Starter Code0:45
04. CODE Building, Running, and Testing2:22
05. Upcoming Exercise and Project Format00:00:48
06. CODE The RouteModel Class0:21
06.2 CODE The RouteModel Class0:21
07. CODE The Node Class00:00:00
08. This Pointer
09. CODE Create RouteModel Nodes0:46
10. CODE Write the Distance Function0:27
11. What Are the Most Important Containers to Learn1:20
12. Hash Tables
13. CODE Create Node to Road Hash Table00:00:00
13.2 CODE Create Node to Road Hash Table2:33
14. CODE Write FindNeighbor00:00:00
14.2 CODE Write FindNeighbor2:31
15. CODE Write FindNeighbors2:22
16. CODE Find the Closest Node0:46
16.2 CODE Find the Closest Node2:15
17. Outro00:00:25
A with OpenStreetMap Data
01. Intro0:19
02. How Long Does it Take to Learn C++00:2:04
03. CODE The RoutePlanner Header00:00:00
04. CODE The RoutePlanner Constructor00:00:00
05. CODE Construct the Final Path0:42
05.2 CODE Construct the Final Path00:2:29
06. CODE Write the A Search Stub1:41
07. CODE Calculate H Values00:00:00
07.2 CODE Calculate H Values0:42
08. CODE Get the Next Node00:00:00
09. CODE Add New Neighbors00:00:00
10. CODE Complete A Search00:00:00
11. CODE Add User Input0:22
11.2 CODE Add User Input0:40
12. Outro0:38
Build an OpenStreetMap Route Planner
01. Intro0:18
02. Submitting Your Project
Project Description – Build an OpenStreetMap Route Planner
Project Rubric – Build an OpenStreetMap Route Planner
Course Outro
01. Outro0:49
02. Career Advice from Bjarne1:23
Welcome
Explore Object-Oriented Programming (OOP) in C++ with examples and exercises covering the essentials of OOP like abstraction and inheritance all the way through to advanced topics like polymorphism and templates. In the end, you’ll build a Linux system monitor application to demonstrate C++ OOP in action!
01. Introduction1:34
02. Creation of C++ and Classes1:37
03. Overview1:25
04. Meet Your Instructor
05. Course Project1:08
06. Let’s Get Started!0:38
Intro to OOP
01. Classes and OOP1:08
02. Classes In C++1:43
03. Jupyter Notebooks
04. Structures2:15
04. Structures Practice00:00:00
05. Member Initialization
06. Access Specifiers4:34
06. Access Specifiers Practice00:00:00
07. Classes1:56
07. Classes Practice00:00:00
08. Encapsulation and Abstraction1:07
09. Encapsulation1:12
10. Constructors00:00:00
10. Constructors Practice00:00:00
11. Scope Resolution1:51
11. Scope Resolution Practice00:00:00
12. Initializer Lists00:00:00
12. Initializer Lists Practice00:00:00
13. Initializing Constant Members3:34
13. Initializing Constant Members Practice00:00:00
14. Encapsulation3:44
14. Encapsulation Practice00:00:00
15. Accessor Functions1:50
15. Accessor Functions Practice00:00:00
16. Mutator Functions00:00:00
16. Mutator Practice00:00:00
18. Exercise Pyramid Class5:44
18. Practice00:00:00
17. Quiz: Classes in C++
19. Exercise Student Class00:00:00
19. Exercise Student Class Practice00:00:00
20. Encapsulation in C++
21. Bjarne On Abstraction00:00:00
22. Abstraction1:24
22. Abstraction00:00:00
23. Exercise Sphere Class00:00:00
23. Exercise Sphere Class Practice00:00:00
24. Exercise Private Method00:00:00
24. Private Method00:00:00
25. Exercise Static Members2:15
25. A second static counter
25. Static Members00:00:00
26. Exercise Static Methods1:28
26. Static Method00:00:00
27. Solving Problems00:00:00
Advanced OOP
01. Polymorphism and Inheritance2:02
02. Inheritance2:46
03. Inheritance
03. Inheritance Practice00:00:00
04. Access Specifiers
04. AccessSpecifiers Practice00:00:00
05. Exercise Animal Class
05. AnimalClass Practice00:00:00
06. Exercise Composition
06. Composition Practice00:00:00
07. Exercise Multiple Inheritance
07. Exercise Multiple Inheritance Practice00:00:00
08. Exercise Class Hierarchy
08. Exercise Class Hierarchy Practice00:00:00
09. Exercise Friends
09. Exercise Friends Practice00:00:00
10. Polymorphism Overloading
10. Polymorphism Overloading Practice00:00:00
11. Exercise Operator Overload
11. Exercise Operator Overload Practice00:00:00
12. Exercise Virtual Functions
12. VirtualFunctions Practice00:00:00
13. Exercise Override
13. Override-1 Practice00:00:00
14. MultipleInheritence Practice00:00:00
15. Generic Programming00:00:00
16. Generic Programming00:00:00
17. Templates
17. Templates Practice00:00:00
18. Templates00:00:00
19. Exercise Comparison Operation
20. Exercise Deduction
21. Exercise Class Template
21. ClassTemplate Practice00:00:00
23. Summary00:00:00
24. Best Practices with Classes00:00:00
Project System Monitor
01. Introduction1:05
02. Project Overview3:28
03. Project Objectives3:16
04. Project File Structure00:3:50
05. Cloud Workspace Intro
06. Project Workspace00:00:00
07. constants.h3:55
08. util.h2:43
08.2 util.h00:00:00
09. ProcessParser.h4:54
10. RAM Usage00:00:00
10.2 RAM Usage4:16
11. CPU Usage00:00:00
12. Process Up Time00:00:00
13. System Up Time00:00:00
14. Process User00:00:00
15. PID List00:00:00
15.2 PID List00:00:00
16. Get Command
17. Number of Cores
18. System CPU Percent00:00:00
19. CPU Stats00:00:00
20. RAM Percent00:00:00
21. System Info00:00:00
21.2 System Info00:00:00
22. Process Class00:00:00
23. Process Container00:00:00
24. Display Info00:00:00
24.2 Display Info4:42
25. Congratulations!00:00:00
26. Bonus ncurses Introduction4:25
27. Bonus ncurses Basic Setup00:00:00
28. Bonus ncurses Print and Modify00:00:00
29. Bonus Exercise ncurses Windowing Part 14:58
30. Bonus Exercise ncurses Windowing Part 21:45
31. Bonus ncurses Windowing Part 17:37
32. Bonus Ncurses Windowing Part 200:00:00
Project Description – System Monitor
Project Rubric – Rubric - System Monitor
Coming Soon Updated System Monitor Project
01. Introduction00:00:00
02. htop2:01
03. Starter Code3:47
04. Goal3:06
05. Project Structure00:00:00
05.2 Project Structure3:40
06. Build Tools2:37
07. System Class00:00:00
08. System Data
09. LinuxParser Namespace00:00:00
09.2 LinuxParser Namespace00:00:00
10. String Parsing00:00:00
11. Processor Class00:00:00
12. Processor Data
13. Process Class00:00:00
14. Process Data
Introduction
Discover the power of memory management in C++ by diving deep into stack vs. heap, pointers, references, new, delete, smart pointers, and much more. By the end, you’ll be ready to work on a chatbot using modern C++ memory management techniques!
01. Introduction00:00:00
02. Memory Management00:00:00
03. RAII00:00:00
04. Stack and Heap00:00:00
05. Bjarne on Stack and Heap00:00:00
06. Stack Memory00:00:00
07. Heap Memory00:00:00
08. C++ in Industry00:00:00
Pointers and References
01. Introduction to Pointers00:00:00
02. Bjarne on pointers00:00:00
03. Pointers Example00:00:00
04. Pointers Exercise 100:00:00
05. Pointers Exercise 200:00:00
06. Pointers Exercise 300:00:00
07. Pointers Exercise 400:00:00
08. Pointers Project Lab00:00:00
09. Introduction to References00:00:00
10. Bjarne on References00:00:00
11. References Example00:00:00
12. References Exercise 100:00:00
new and delete
01. Introduction00:00:00
02. Bjarne on new and delete00:00:00
03. new
04. new – Example00:00:00
05. delete
06. delete – Example1:22
07. new and delete Exercise 100:00:00
08. new and delete Exercise 200:00:00
10. new and delete Project Exercise00:00:00
11. Memory Leaks00:00:00
12. memset and malloc00:00:00
13. memset00:00:00
14. memset – Example00:00:00
15. malloc00:00:00
16. malloc – Example00:00:00
Smart Pointers
01. Bjarne on Smart Pointer00:00:00
02. Bjarne on Pointer Best Practices00:00:00
03. Smart Pointers1:57
04. Smart Pointer Exercise 100:00:00
05. Smart Pointer Exercise 200:00:00
06. Resource Scope
07. Bjarne on Scope0:48
08. Resource Scope Exercise 1
09. Resource Scope Exercise 2
10. Bjarne on Importance of Scope2:04
Garbage Collector
01. Project Introduction0:27
02. Project Overview
03. Pointer Lab
04. Reference Lab
05. New and Delete Lab
06. Smart Pointer Explanation
07. Smart Pointer Lab
08. Resource Scope Lab
09. Garbage Collector
10. Project Workspace00:00:00
Project Description – Garbage Collector
Project Rubric – Garbage Collector
Introduction and Running Threads
Concurrent programming runs multiple threads of execution in parallel. Concurrency is an advanced programming technique that, when properly implemented, can dramatically accelerate your C++ programs.
01. Introduction00:00:00
02. Processes and Threads00:00:00
02. Processes and Threads
02.2 Processes and Threads00:00:00
03. Running a Single Thread00:00:00
03.2 Running a Single Thread00:00:00
03. Running a Single Thread Practice00:00:00
04. Starting a Thread with Function Objects00:00:00
04.2 Starting a Thread with Function Objects00:00:00
04.3 Starting a Thread with Function Objects00:00:00
04. Starting a Thread with Function Objects Practice00:00:00
05. Starting a Thread with Variadic Templates & Member Functions00:00:00
05.2 Starting a Thread with Variadic Templates & Member Functions00:00:00
05. Starting a Thread with Variadic Templates & Member Functions Practice00:00:00
06. Running Multiple Threads00:00:00
06.2 Running Multiple Threads00:00:00
06. Running Multiple Threads Practice00:00:00
07. Lesson 1 Exercise00:00:00
07.2 Lesson 1 Exercise00:00:00
Passing Data Between Threads
01. Introduction00:00:00
02. Promises and Futures00:00:00
02.2 Promises and Futures00:00:00
02. Promises and Futures Practice00:00:00
03. Threads vs. Tasks00:00:00
03. Threads vs. Tasks Practice00:00:00
04. Threads vs. Tasks Quiz00:00:00
04. Threads vs. Tasks Quiz
05. Avoiding Data Races00:00:00
05.2 Avoiding Data Races00:00:00
05. Avoiding Data Races Practice00:00:00
06. Lesson 2 Exercise00:00:00
06.2 Lesson 2 Exercise00:00:00
Mutexes and Locks
01. Introduction00:00:00
02. Using a Mutex to Protect Shared Data00:00:00
02.2 Using a Mutex to Protect Shared Data00:00:00
02.3 Using a Mutex to Protect Shared Data00:00:00
02. Using a Mutex to Protect Shared Data Practice00:00:00
03. Using Locks to Avoid Deadlocks00:00:00
03.2 Using Locks to Avoid Deadlocks00:00:00
03. Using Locks to Avoid Deadlocks00:00:00
04. Lesson 3 Exercise00:00:00
04.2 Lesson 3 Exercise00:00:00
Condition Variables and Message Queues
01. Introduction0:56
02. The Monitor Object Pattern00:00:00
02.2 The Monitor Object Pattern00:00:00
03. Building a Concurrent Message Queue00:00:00
03.2 Building a Concurrent Message Queue00:00:00
02. The Monitor Object Pattern Practice00:00:00
03. Building a Concurrent Message Queue Practice00:00:00
Program a Concurrent Traffic Simulation
01. Final Project Intro0:47
02. Final Project Tasks11:28
03. Final Project Code Overview00:00:00
04. Final Project Workspace00:00:00
Project Rubric – Program a Concurrent Traffic Simulation
Project Description – Program a Concurrent Traffic Simulation
Industry Research
01. Self-Reflection Design Your Blueprint for Success
01. Self-Reflection: Design Your Blueprint for Success
02. Debrief Self-Reflection Exercise Part 1
03. Debrief Self-Reflection Exercise Part 2
04. Map Your Career Journey
05. Debrief Map Your Career Journey
06. Conduct an Informational Interview
07. How to Request an Informational Interview
07. How to Request an Informational Interview
08. Ways to Connect
09. Ask Good Questions
09. Ask Good Questions
10. Debrief Sample Questions Quiz
11. Keep the Conversation Going
Capstone —
Put your C++ skills to use on a project of your own! You’ll utilize the core concepts from this Nanodegree program - object-oriented programming, memory management, and concurrency - to build your own application using C++.
01. Capstone Intro2:36
02. Steps to Complete the Capstone00:00:00
03. Game Programming00:00:00
03.1 Game Programming
03.2 Game Programming00:00:00
03.2 Game Programming
04. The SDL Library00:00:00
05. Snake Game Code Walkthrough00:00:00
05.2 Snake Game Code Walkthrough00:00:00
05.3 Snake Game Code Walkthrough00:00:00
05.4 Snake Game Code Walkthrough00:00:00
05.5 Snake Game Code Walkthrough00:00:00
06. Capstone Workspace
Project Description – C++ Capstone Project
Project Rubric – C++ Capstone Project
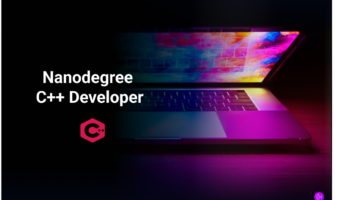